Software Engineering in the D Programming Language - A Tour of DLang for your Competitive Advantage
Mike Shah
09:00-18:00, Saturday, 12th April 2025
The following hands-on training provides a tour of the essential parts of the mature and multi-paradigm programming language D. In this workshop attendees will learn about the programming language paradigms supported in D, core idioms, and the essential features that allow writing ‘better code’ the default option in the D programming language. This workshop will include hands-on exercises that enable attendees to practice as they learn during the workshop (i.e. the workshop will be broken into ~5 modules each an hour long with 45 minutes of lecture, followed by 15 minutes of practice, and then a summary and short break before the next module). Attendees should have experience programming in at least one language (e.g. C, C++, Java, Go, Rust, etc.), but are not required to have any D programming language experience. Regardless if you end up using D in your daily programming or as a hobby, attendees will leave this training better understanding idioms in concurrency, and otherwise how to think about programming.
Outline
- Course introduction - Introduction to instructor, learning objectives, and how to succeed.
- Resources on how to learn and strategies for retaining information will be implemented by attendees.
- Acquiring D Compilers
- D Tour - A quick tour of the language and the big ideas
- Core ideas of the D programming language
- Better and more sensible defaults, multi-paradigms, opt-out memory safety
- Compile-time versus run-time
- Understanding Compile-time Function Execution (CTFE), static if, static foreach, and mixins.
- Core Language Features
- arrays/slices/associative arrays
- What is a slice
- arrays/slices/associative arrays
- functions
- Uniform Function Call Syntax (UFCS)
- lambdas, function pointers and delegates
- structs, classes and interfaces
- Value Type versus Reference Type Semantics
- ranges
- templates
- Understanding when and where to apply meta-programming techniques.
- Uniform Function Call Syntax (UFCS)
- Activity:
- Building a small json parser.
- Core ideas of the D programming language
- DLang Batteries Included - Phobos Standard Library
- Data Structures and Containers
- Iterators/Ranges
- Algorithm
- Activity:
- Using D’s profiler
- DLang Object Oriented Programming - Project
- In this section we see DLang in action building a ‘ray tracer’
- Students will learn about how to design a ray tracer utilizing multiple features of the language.
- Briefly I will also show features along the way regarding
- Object-oriented design
- Utilizing maps and arrays
- Serialization of data with json
- Compression of result with zlib
- Concurrency in D
- std.parallel to boost performance
- Utilizing Message Passing for a work queue
- Unit testing with:
- -unittest
- DDoc - For documentation
- Dub - The D package manager
- DLang Selected Topics - Further topics with examples
- Depending on time and a survey of attendees interests the following topics will be covered more/less in depth to end the workshop.
- Attributes in DLang
- Memory Safety in the D Programming Language
- Discussion of how to avoid the garbage collection (@ nogc
- DIP1000 (in, out, return, return scope)
- @safe attribute and escape analysis to avoid memory errors.
- @live -- the ‘borrow checker’ (similar to Rust)
- Operator Overloading in DLang
- Contract-Based Programming
- importC
- Interop with C, C++, and Obj-C
- Synchronization (Message Passing, Fibers, and Parallelism)
- The Vibe.d project for networking and backend development
- Dependency management in D
- DLang Closing Notes
- Q&A
- Where to continue learning
- How to get involved in the community
Mike Shah
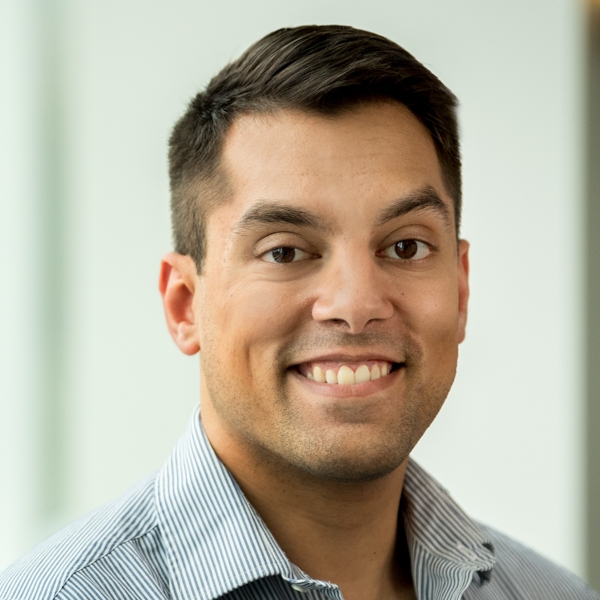
Mike Shah is an Associate Teaching Professor at Northeastern University in the Khoury College of Computer Sciences. His primary teaching interests are in computer systems, computer graphics, and software engineering. His research interests are related to performance engineering (dynamic analysis), software visualization, and computer graphics. Along with teaching and research work, he have juggled occasional consulting work as a 3D Senior Graphics Engineer in C++. Mike enjoys creating programming content at https://youtube.com/c/MikeShah